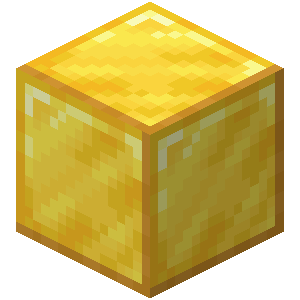
Jobs Reborn Menu - EN/PT 1.0
Easy to use and understand Jobs Reborn menu with 12 jobs with levels, payouts and more
Jobs Menu for Jobs Reborn using Deluxe Menus, configuration includes 12 jobs with a level system with rewards, description of the profession and the actions that each one has to receive payments
Clean and objective menu, your players won't stop wanting to work!
Check some images:
Main menu
Main Menu | Personal Statistics
Woodcutter job | Not working on job
Woodcutter Job | Working + player stats
Woodcutter Job | Levels Menu
Woodcutter Job | Levels Menu | Click to claim reward
Woodcutter Job | Levels Menu | Can't claim
Installation:
1. After purchasing download the zip, and unpack it;
2. Download: Jobs Reborn and it dependence which is necessary for it to work, Deluxe Menus and the PlaceholderAPI plugin;
3. Drop the "jobs" menu folder into the Deluxe Menus' menus folder
4. Start your server